In today’s fast-paced digital world, efficiency is key. Whether you’re a developer, system administrator, or just a tech-savvy individual, automating repetitive tasks can save you a significant amount of time and effort. One of the most powerful yet underrated tools for automation on Windows is the humble batch file. Batch files allow you to automate a series of commands, making your workflow smoother and more efficient. In this comprehensive guide, we’ll explore how you can leverage batch files to automate everything, with 10 practical examples to get you started.
1. Introduction to Batch Files
Batch files, also known as batch scripts or command scripts, are text files containing a series of commands that are executed by the command-line interpreter on Windows. These files have a .bat
or .cmd
extension and are used to automate repetitive tasks, making them an invaluable tool for anyone looking to streamline their workflow.
Batch files have been around since the early days of DOS (Disk Operating System) and continue to be relevant today. They are simple to create, easy to modify, and can be executed on any Windows machine without the need for additional software.
2. Why Automate with Batch Files?
2.1. Time-Saving
One of the most significant advantages of using batch files is the time they save. By automating repetitive tasks, you can focus on more important aspects of your work. For example, instead of manually copying files from one directory to another every day, you can create a batch file to do it for you with a single click.
2.2. Consistency
Automation ensures that tasks are performed consistently every time. Human error is inevitable, but batch files execute commands exactly as they are written, reducing the risk of mistakes.
2.3. Flexibility
Batch files are highly flexible. You can use them to perform a wide range of tasks, from simple file operations to complex system management. They can also be combined with other scripting languages and tools to create even more powerful automation solutions.
2.4. Accessibility
Batch files are easy to create and require no special software. All you need is a text editor like Notepad, and you’re ready to go. This makes them accessible to anyone, regardless of their technical expertise.
3. Getting Started with Batch Files
Before diving into the examples, let’s cover the basics of creating and running batch files.
3.1. Creating a Batch File
- Open Notepad or any text editor.
- Write your commands, one per line.
- Save the file with a
.bat
or.cmd
extension (e.g.,backup.bat
).
3.2. Running a Batch File
- Double-click the batch file to execute it.
- Alternatively, open Command Prompt and navigate to the directory containing the batch file, then type the file name and press Enter.
3.3. Basic Commands
Here are some basic commands you’ll frequently use in batch files:
echo
: Displays messages or turns command echoing on/off.@echo off
: Hides the command prompt output.rem
: Adds comments (remarks) to your script.pause
: Pauses the script and waits for user input.exit
: Closes the Command Prompt window.
Now that you’re familiar with the basics, let’s move on to the practical examples.
4. 10 Practical Examples of Batch File Automation
Example 1: Automating File Backups
Scenario: You want to back up important files from one directory to another every day.
Solution:
@echo off echo Backing up files... xcopy "C:\ImportantFiles\*.*" "D:\Backup\ImportantFiles\" /s /e /h /y echo Backup complete! pause
Explanation:
xcopy
is used to copy files and directories./s
copies directories and subdirectories, except empty ones./e
copies all subdirectories, including empty ones./h
copies hidden and system files./y
suppresses prompting to confirm overwriting a file.
Example 2: Cleaning Up Temporary Files
Scenario: You want to delete temporary files to free up disk space.
Solution:
@echo off echo Cleaning up temporary files... del /q/f/s %TEMP%\* echo Temporary files deleted! pause
Explanation:
del
deletes files./q
enables quiet mode (no confirmation)./f
forces deletion of read-only files./s
deletes specified files from all subdirectories.
Example 3: Automating Software Installation
Scenario: You want to install multiple software applications silently.
Solution:
@echo off echo Installing software... start /wait msiexec /i "C:\Software\App1.msi" /quiet start /wait msiexec /i "C:\Software\App2.msi" /quiet echo Software installation complete! pause
Explanation:
start /wait
runs a program and waits for it to finish before proceeding.msiexec /i
installs an MSI package./quiet
performs a silent installation with no user interaction.
Example 4: Scheduling Tasks with Batch Files
Scenario: You want to schedule a batch file to run at a specific time.
Solution:
- Create a batch file (e.g.,
backup.bat
). - Open Task Scheduler.
- Create a new task and set the trigger to the desired time.
- Set the action to start the batch file.
Batch File:
@echo off echo Running scheduled backup... xcopy "C:\ImportantFiles\*.*" "D:\Backup\ImportantFiles\" /s /e /h /y echo Scheduled backup complete!
Example 5: Automating File Renaming
Scenario: You want to rename all .txt
files in a directory.
Solution:
@echo off echo Renaming files... for %%f in (*.txt) do ( ren "%%f" "NewName_%%f" ) echo Files renamed! pause
Explanation:
for %%f in (*.txt)
loops through all.txt
files in the directory.ren
renames the files.
Example 6: Batch File for System Information
Scenario: You want to gather system information and save it to a file.
Solution:
@echo off echo Gathering system information... systeminfo > systeminfo.txt echo System information saved to systeminfo.txt! pause
Explanation:
systeminfo
displays detailed system information.>
redirects the output to a file.
Example 7: Automating Network Commands
Scenario: You want to ping multiple devices on your network.
Solution:
@echo off echo Pinging network devices... ping 192.168.1.1 > ping_results.txt ping 192.168.1.2 >> ping_results.txt ping 192.168.1.3 >> ping_results.txt echo Ping results saved to ping_results.txt! pause
Explanation:
ping
sends ICMP echo requests to a network device.>
and>>
redirect output to a file (>
overwrites,>>
appends).
Example 8: Creating a Simple Menu System
Scenario: You want to create a menu for common tasks.
Solution:
@echo off :menu cls echo 1. Backup Files echo 2. Clean Temporary Files echo 3. Exit set /p choice=Choose an option: if "%choice%"=="1" goto backup if "%choice%"=="2" goto clean if "%choice%"=="3" exit goto menu :backup echo Backing up files... xcopy "C:\ImportantFiles\*.*" "D:\Backup\ImportantFiles\" /s /e /h /y echo Backup complete! pause goto menu :clean echo Cleaning up temporary files... del /q/f/s %TEMP%\* echo Temporary files deleted! pause goto menu
Explanation:
:menu
,:backup
, and:clean
are labels.set /p
prompts the user for input.if
statements handle user choices.
Example 9: Automating File Transfers
Scenario: You want to transfer files to a remote server using FTP.
Solution:
@echo off echo Transferring files to remote server... ftp -s:ftp_commands.txt echo File transfer complete! pause
ftp_commands.txt:
open ftp.example.com username password cd /remote/directory lcd C:\Local\Directory mput *.txt quit
Explanation:
ftp -s
runs FTP commands from a script file.open
connects to the FTP server.cd
changes the remote directory.lcd
changes the local directory.mput
uploads multiple files.
Example 10: Batch File for Shutdown or Restart
Scenario: You want to shut down or restart your computer with a single click.
Solution:
@echo off echo 1. Shutdown echo 2. Restart set /p choice=Choose an option: if "%choice%"=="1" shutdown /s /t 0 if "%choice%"=="2" shutdown /r /t 0
Explanation:
shutdown /s
shuts down the computer.shutdown /r
restarts the computer./t 0
sets the time delay to 0 seconds.
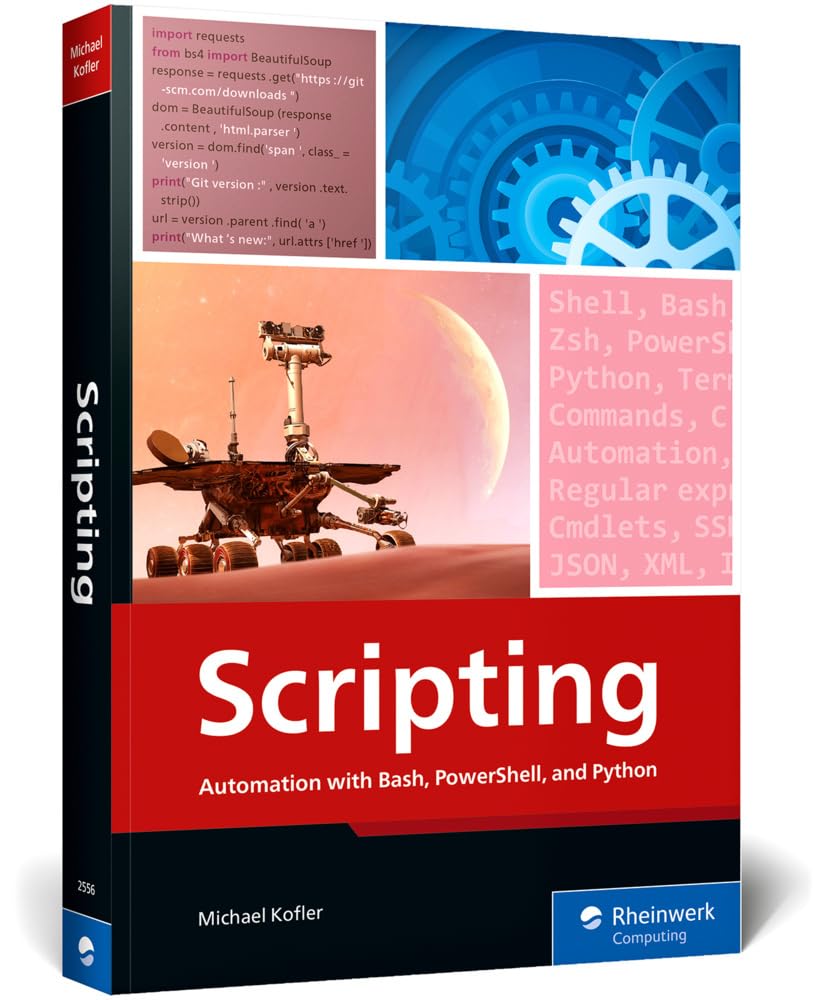
Scripting: Automation with Bash, PowerShell, and Python—Automate Everyday IT Tasks from Backups to Web Scraping in Just a Few Lines of Code.
5. Best Practices for Writing Batch Files
- Use Comments: Add comments (
rem
) to explain the purpose of each section of your script. - Test Thoroughly: Always test your batch files in a safe environment before deploying them.
- Error Handling: Include error handling to manage unexpected issues.
- Keep It Simple: Avoid overly complex scripts; break them into smaller, manageable files if necessary.
- Use Variables: Use variables to make your scripts more flexible and easier to maintain.
6. Conclusion
Batch files are a powerful yet often overlooked tool for automating tasks on Windows. By mastering batch scripting, you can save time, reduce errors, and streamline your workflow. The examples provided in this guide are just the tip of the iceberg—batch files can be used for a wide range of tasks, from simple file operations to complex system management.
Whether you’re a beginner or an experienced user, batch files offer a simple and effective way to automate your daily tasks. So why not give them a try? Start with the examples provided, and soon you’ll be creating your own custom scripts to automate everything.
By following this guide, you’ll be well on your way to becoming a batch file pro. Remember, the key to successful automation is to start small, test thoroughly, and gradually build up your skills. Happy scripting!
See Also
-
Windows 11 Performance Hacks: Stop Background Apps from Wasting Your Power!
-
No Internal Storage? No Problem! Install Windows 11 on an External SSD Today
-
Save Time & Effort: Automate Everything with Batch Files
-
Mastering Microsoft Intune: Revolutionize Your Device Management Strategy Today!
-
The Ultimate Guide to Securing Your System with BitLocker, Windows Defender, and Firewall Rules
-
The Ultimate Guide to Troubleshooting Advanced System Issues in Windows 11
-
Revolutionize Your Digital Life: The Ultimate Guide to Cloud Integration in Windows 11